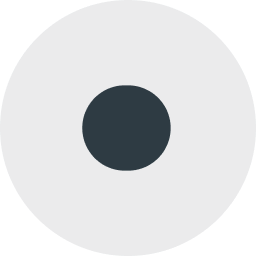

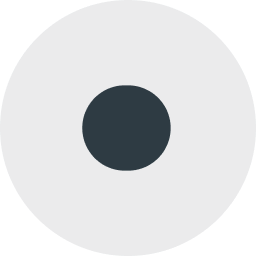

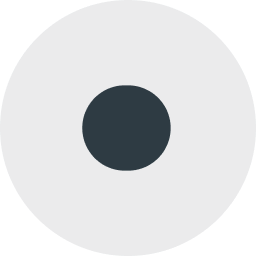



Expand your analysis with the new CARTO Workflows Extensions
Expand & simplify your spatial analytics with AI-powered CARTO Workflows Extension Packages. Automate tasks, ensure consistency & enhance geospatial insights!
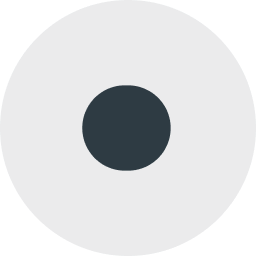

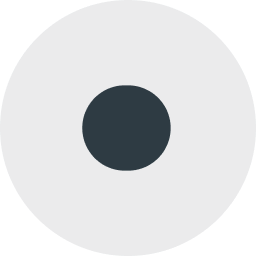
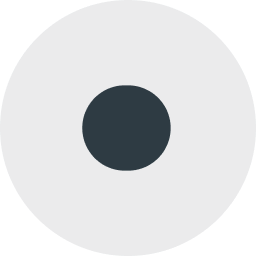

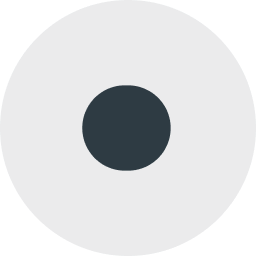
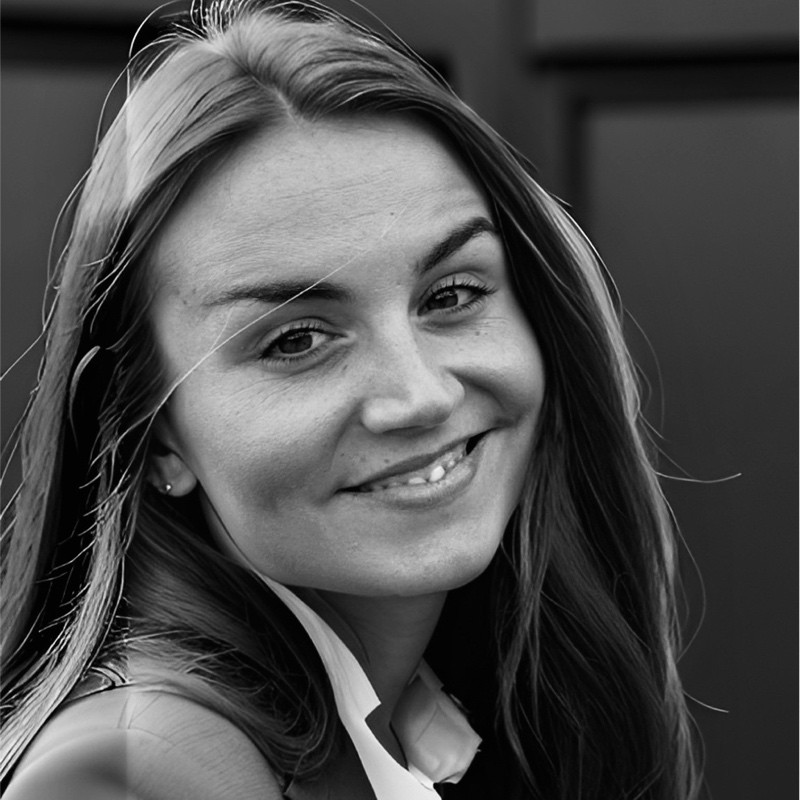
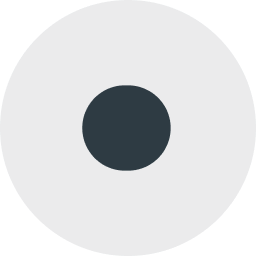

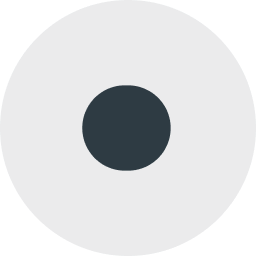
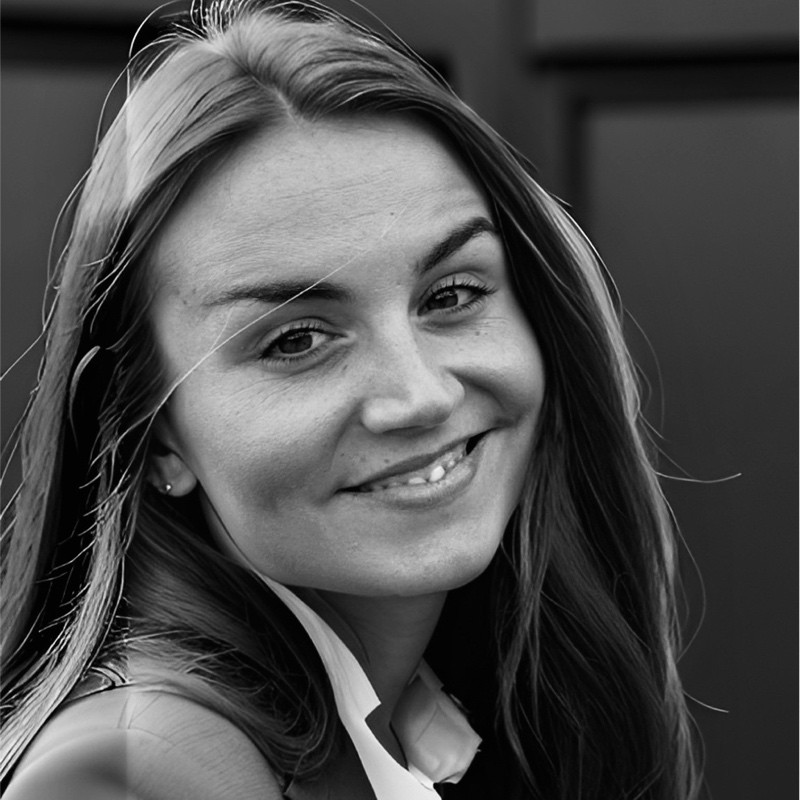
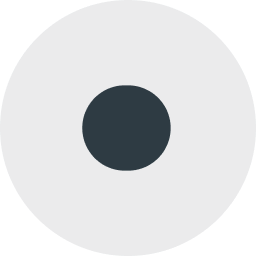
Drive smarter decisions with new BT mobility data in CARTO
Unlock mobility insights with BT Active Intelligence & CARTO! Drive smarter decisions with road-level data, OD matrices & seamless cloud integration.
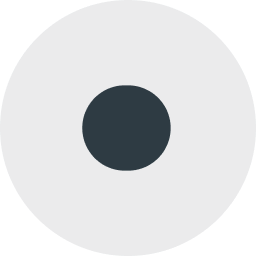

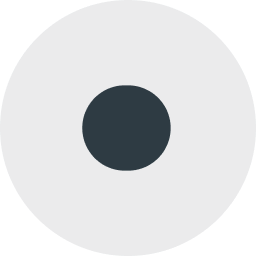
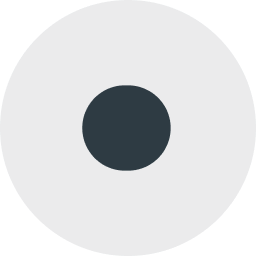

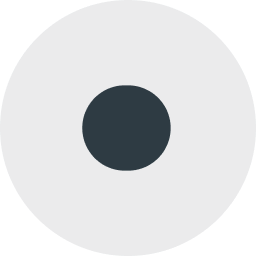

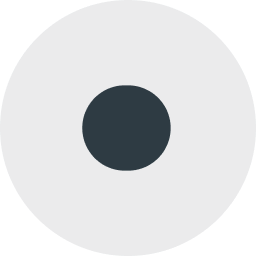

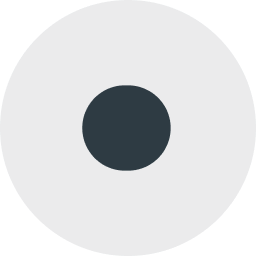

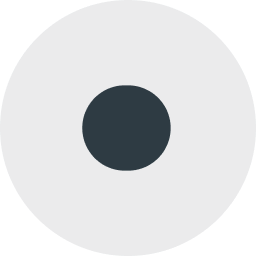
What's New in CARTO - Q4 2024
Announcing the latest new features in the CARTO platform from Q4 2024! Explore the latest in visualisation, app development, analytics & security.
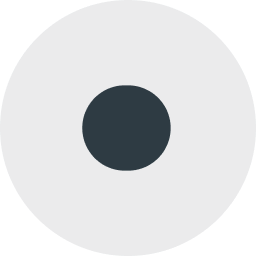

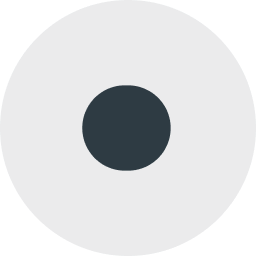
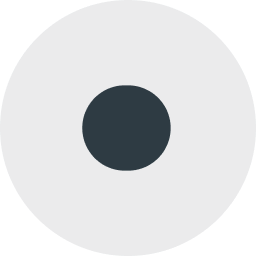

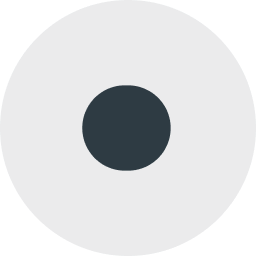
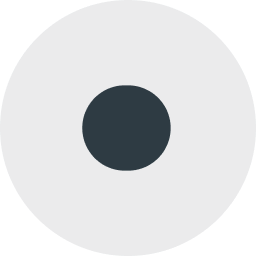

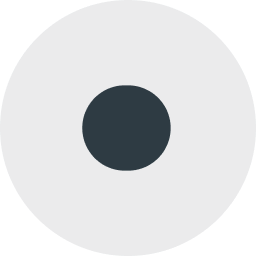
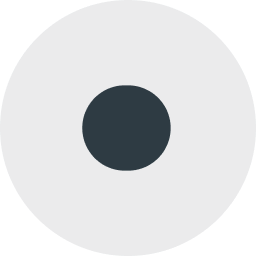
24 of the best maps, visualizations & analysis from 2024
Discover 24 of the best maps, visualizations, and analyses from 2024! Explore innovations in geospatial data, user-centric tools, & advanced spatial analytics.
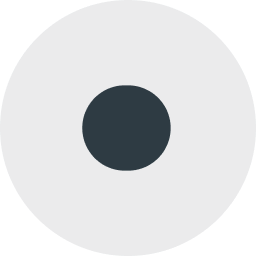

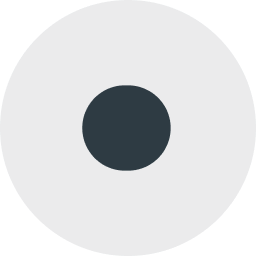
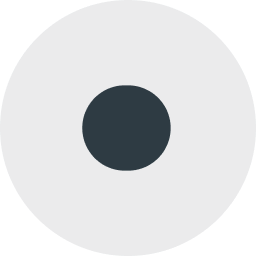

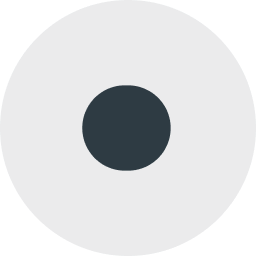
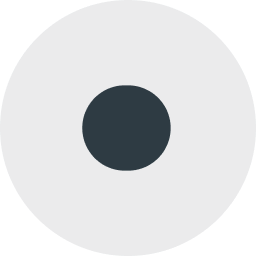

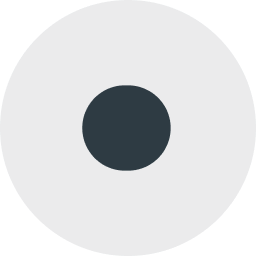
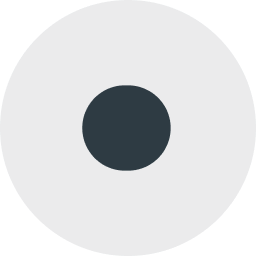
Deploy CARTO inside Snowflake using Container Services
Discover the power of spatial analytics with CARTO inside Snowflake! Deploy seamlessly via Snowflake Marketplace for enhanced performance, security, and scalability.
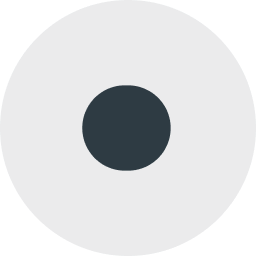

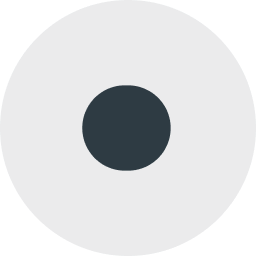
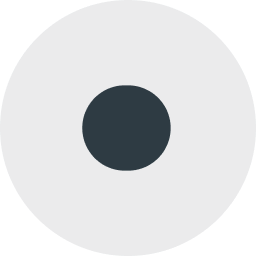

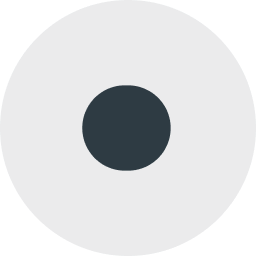
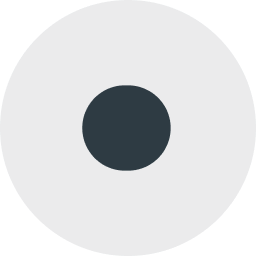

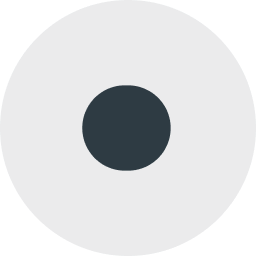
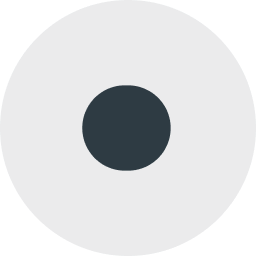
Embed your maps in Power BI, Tableau or your favorite BI tool
Learn how to embed CARTO maps in Power BI, Tableau, and other BI tools to enhance dashboards with interactive spatial data visualization and insights.
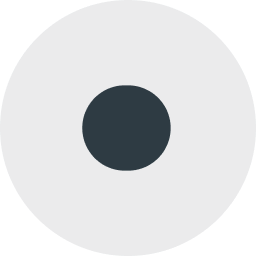

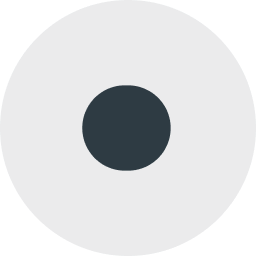
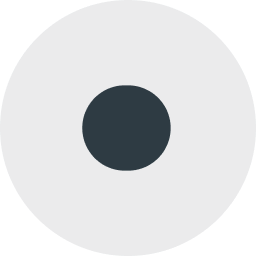

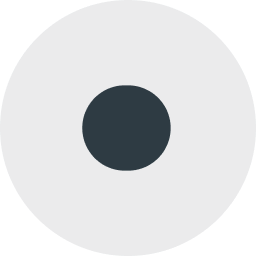
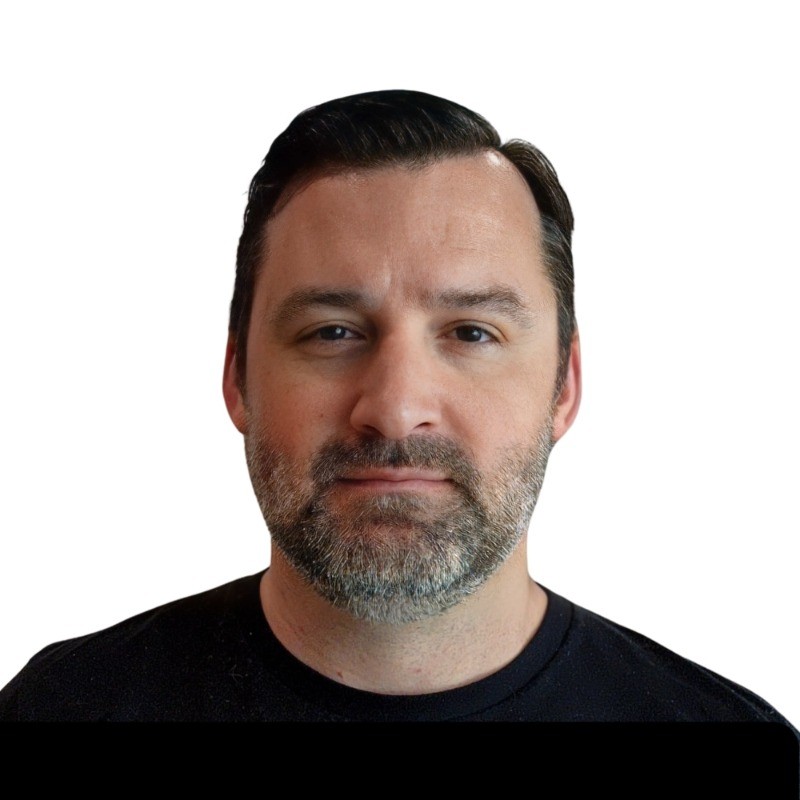
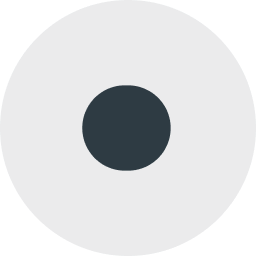

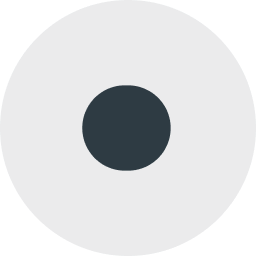
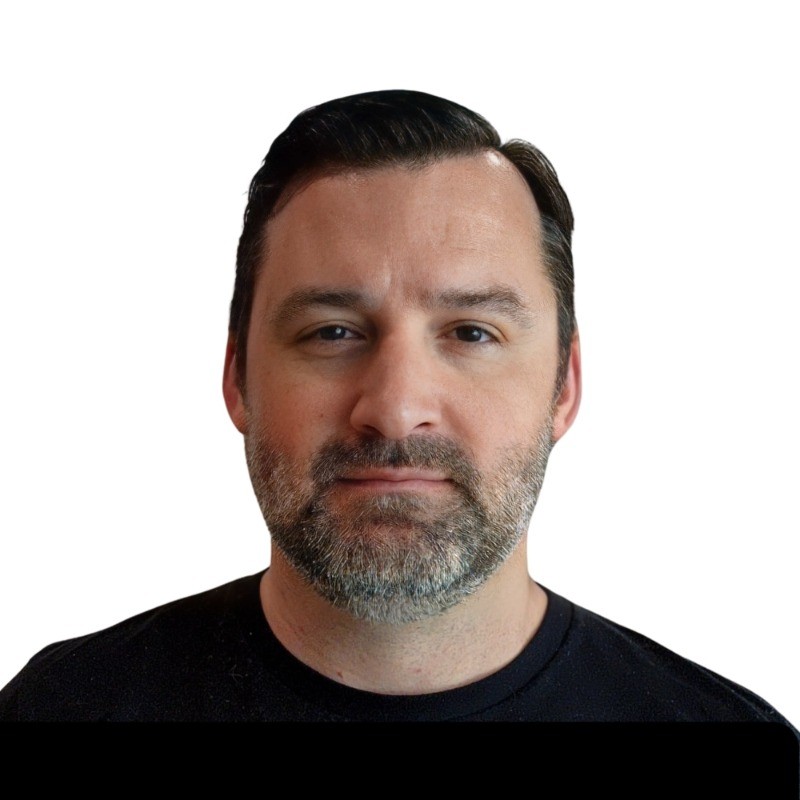
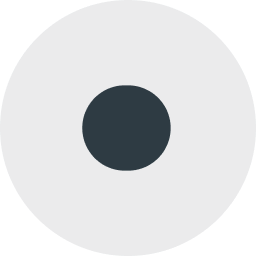
CARTO for Databricks: True Native Geospatial for the Lakehouse
Discover CARTO's integration with Databricks, empowering users with native geospatial analytics for enhanced Location Intelligence and streamlined workflows in the Lakehouse.
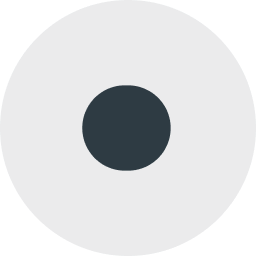
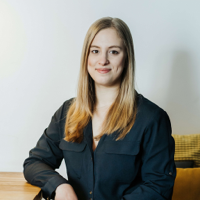
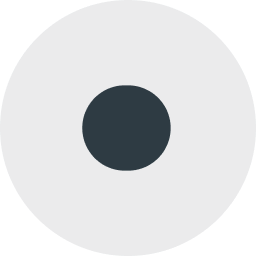
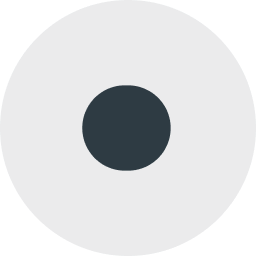
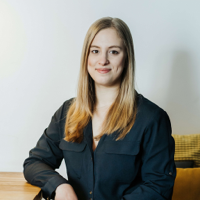
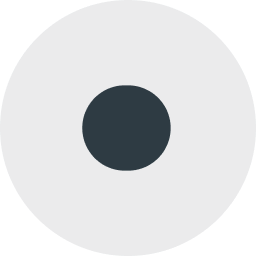
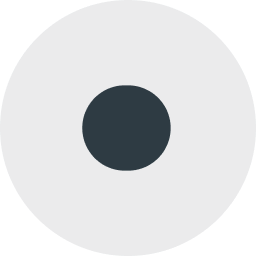
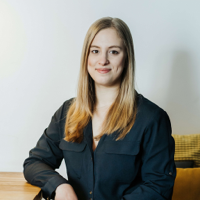
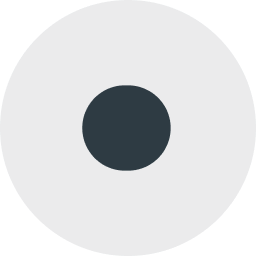
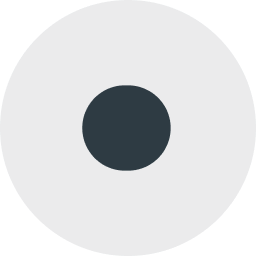
Recap of SDSC24 NYC & CARTO’s Vision for the Future
Latest innovations in geospatial analytics from CARTO, industry insights, and AI-driven spatial tools showcased at the Spatial Data Science Conference in NYC.
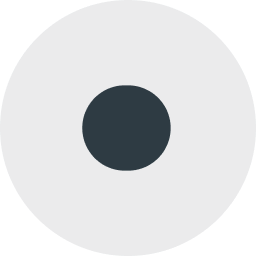

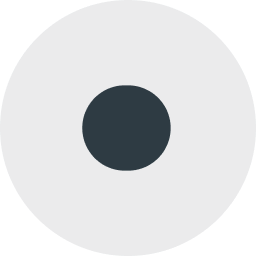
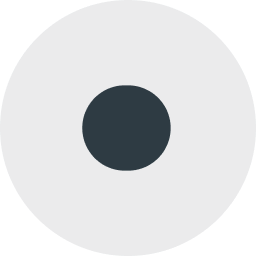

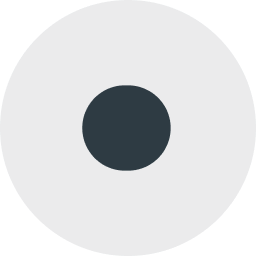

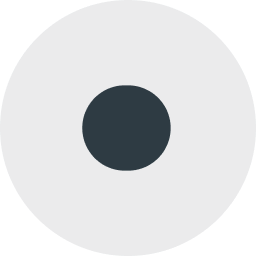

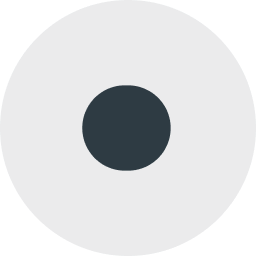

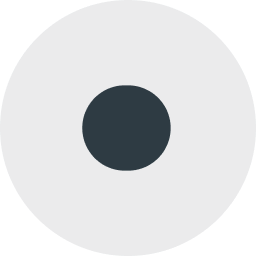
What's New in CARTO - Q3 2024
Announcing the latest new features in the CARTO platform from Q3 2024.
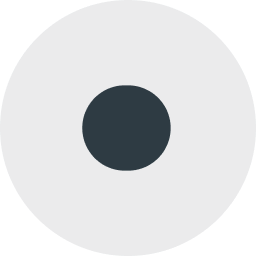
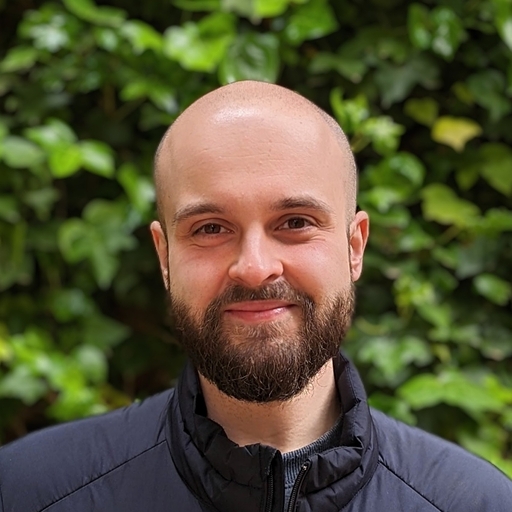
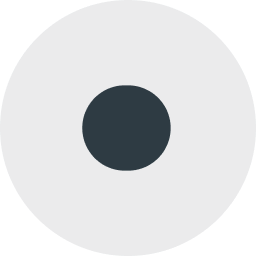
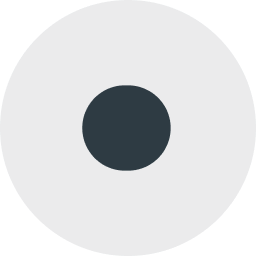
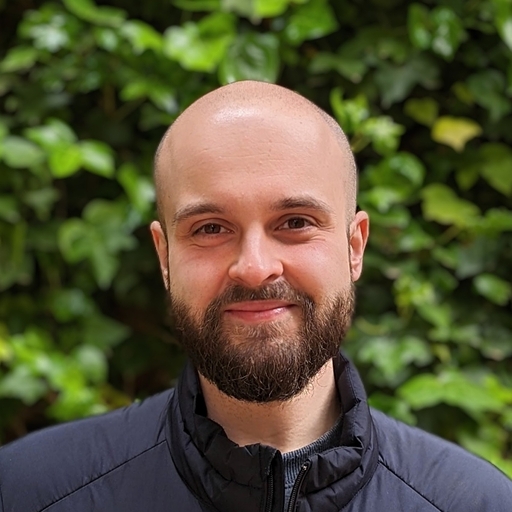
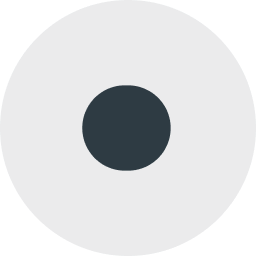

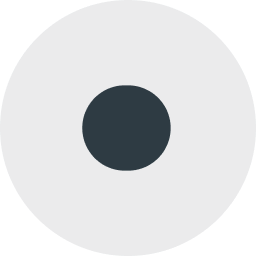
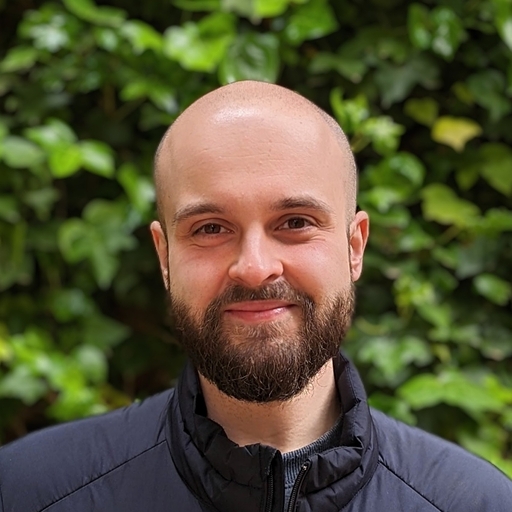
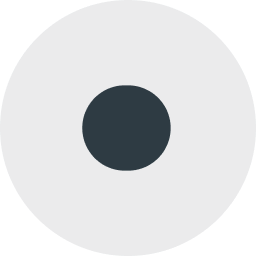

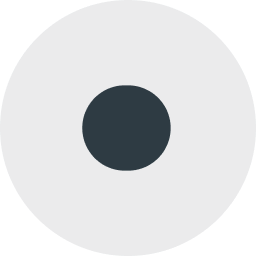
What space-time analysis tells us about the Paris Olympics
Explore space-time analytics through the 2024 Paris Olympics! Discover insights from human mobility data & learn how to leverage spatial analysis tools.
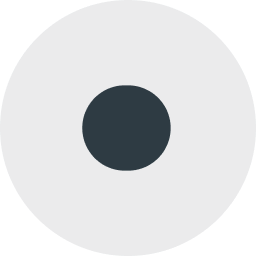

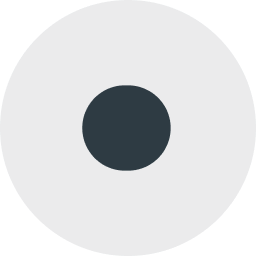
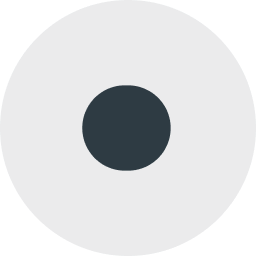

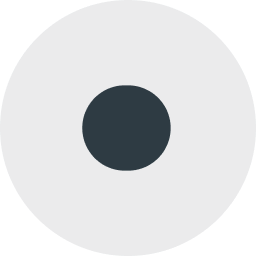
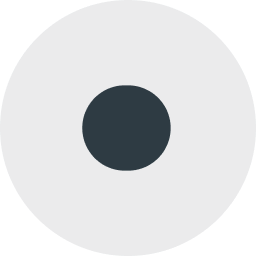

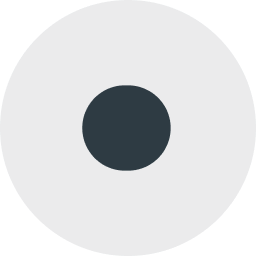
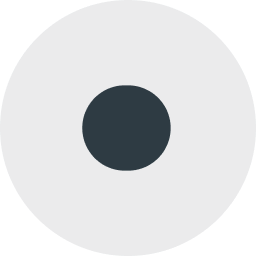
Spatial analysis & visualization for more intelligent tourism planning
Discover how spatial data analysis and visualization empowers tourism organizations to personalize experiences, optimize resources & make strategic decisions.
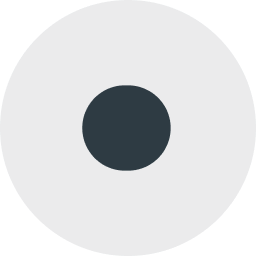

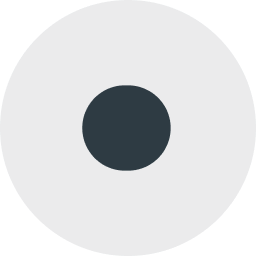
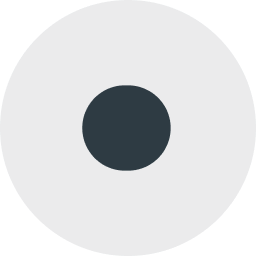

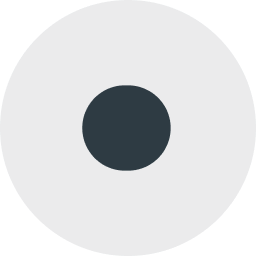

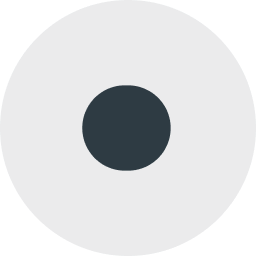

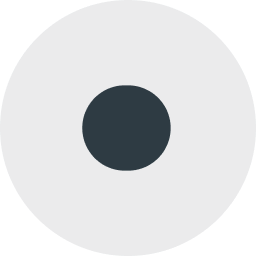

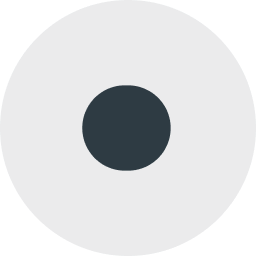
Urban Mobility Insights with MovingPandas & CARTO in Snowflake
Learn how integrating MovingPandas with CARTO in Snowflake boosts urban mobility analysis by uncovering traffic hotspots and optimizing city transportation.
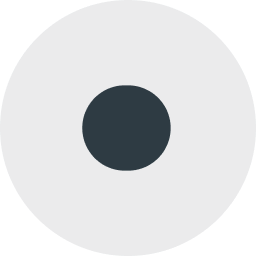
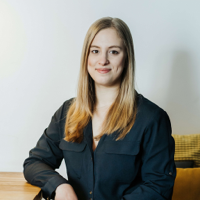
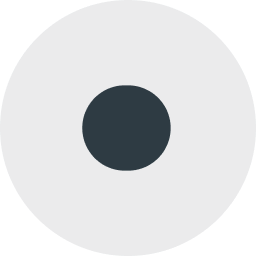
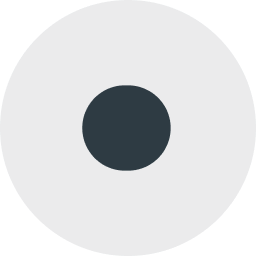
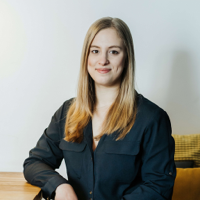
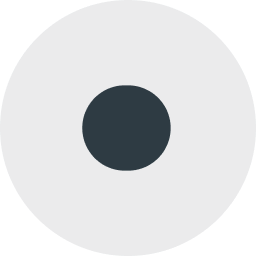
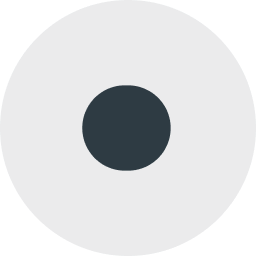
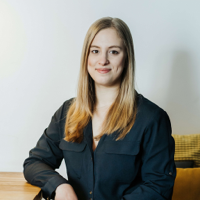
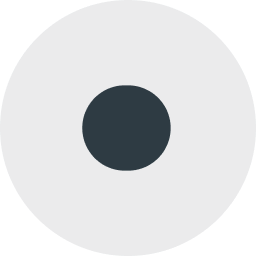
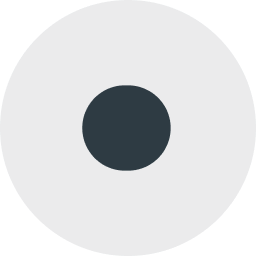
Discover the Future of Spatial Data Science at SDSC24 New York
Discover the latest in Spatial Data Science at SDSC24 New York! Join us on October 16-17 for insights, workshops, and networking opportunities.
Location intelligence resources straight to your inbox
Subscribe to our latest news, product features and events.
Academy
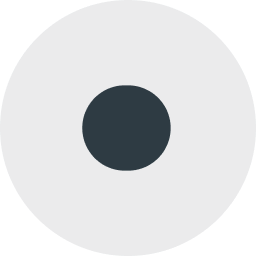

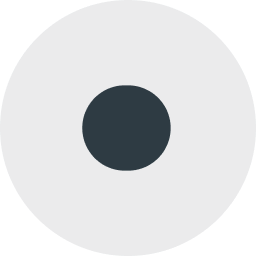

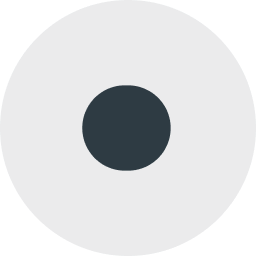

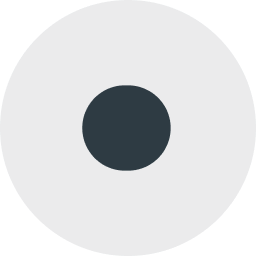

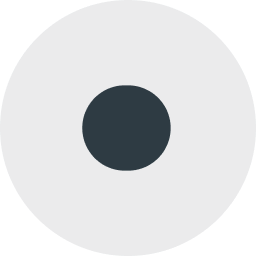

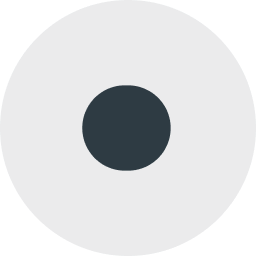
Expand your analysis with the new CARTO Workflows Extensions
Expand & simplify your spatial analytics with AI-powered CARTO Workflows Extension Packages. Automate tasks, ensure consistency & enhance geospatial insights!
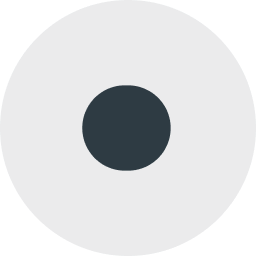

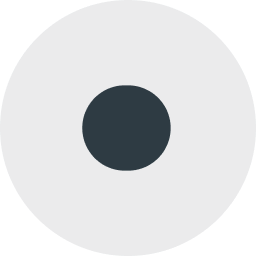

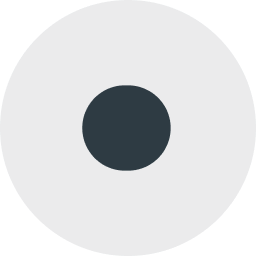
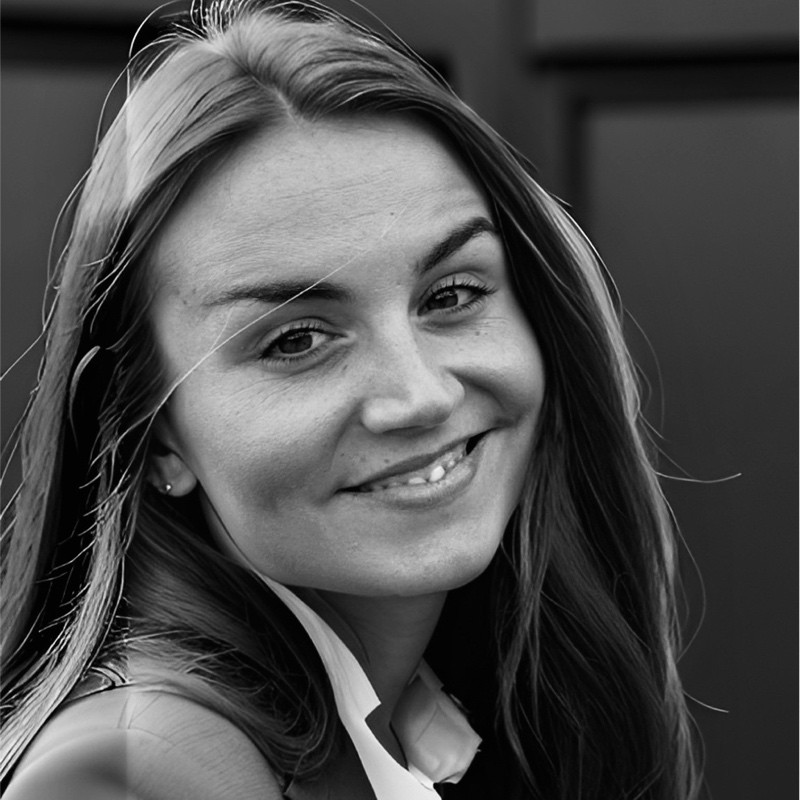
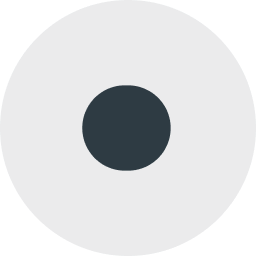

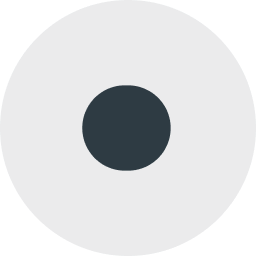
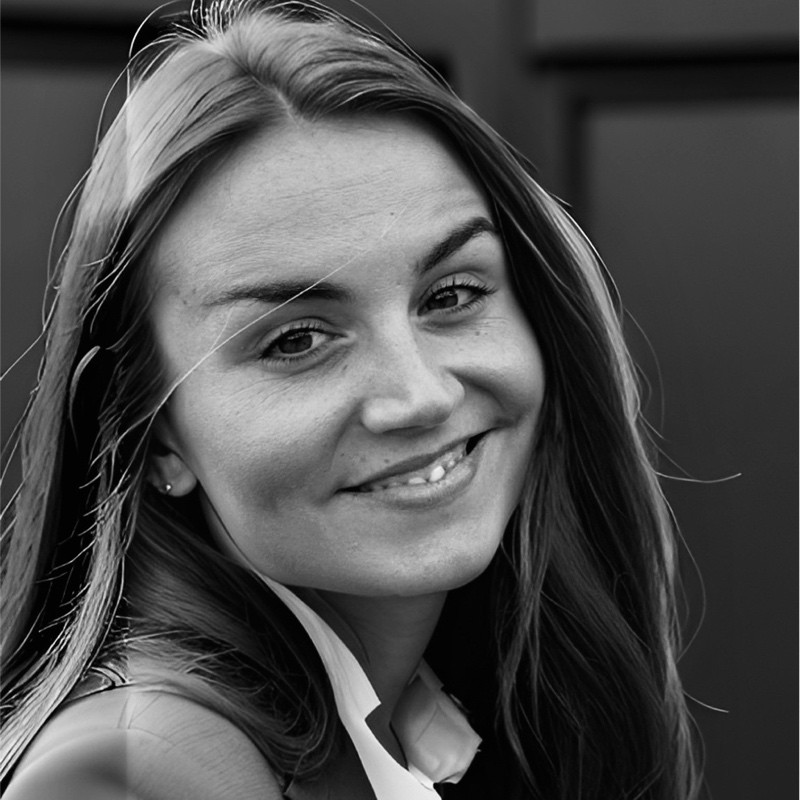
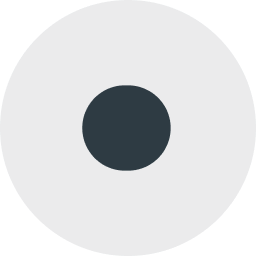
Drive smarter decisions with new BT mobility data in CARTO
Unlock mobility insights with BT Active Intelligence & CARTO! Drive smarter decisions with road-level data, OD matrices & seamless cloud integration.
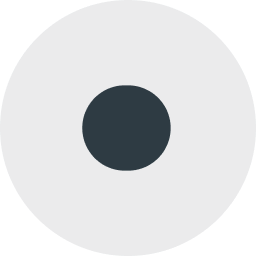

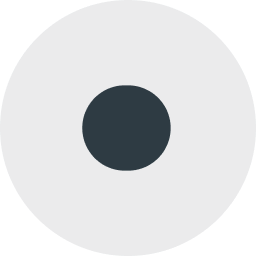

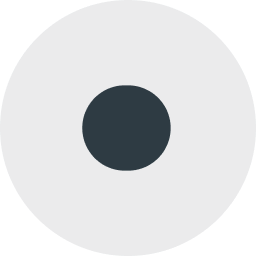

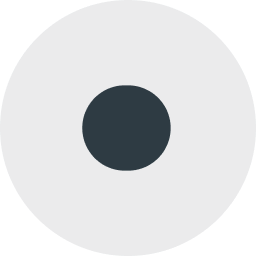

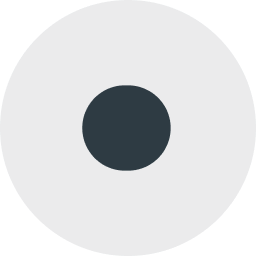

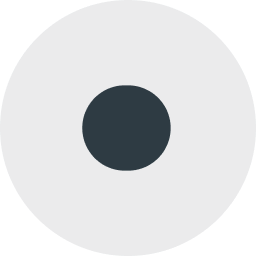
What's New in CARTO - Q4 2024
Announcing the latest new features in the CARTO platform from Q4 2024! Explore the latest in visualisation, app development, analytics & security.