How to use Angular & CARTO to Build Scalable Spatial Apps
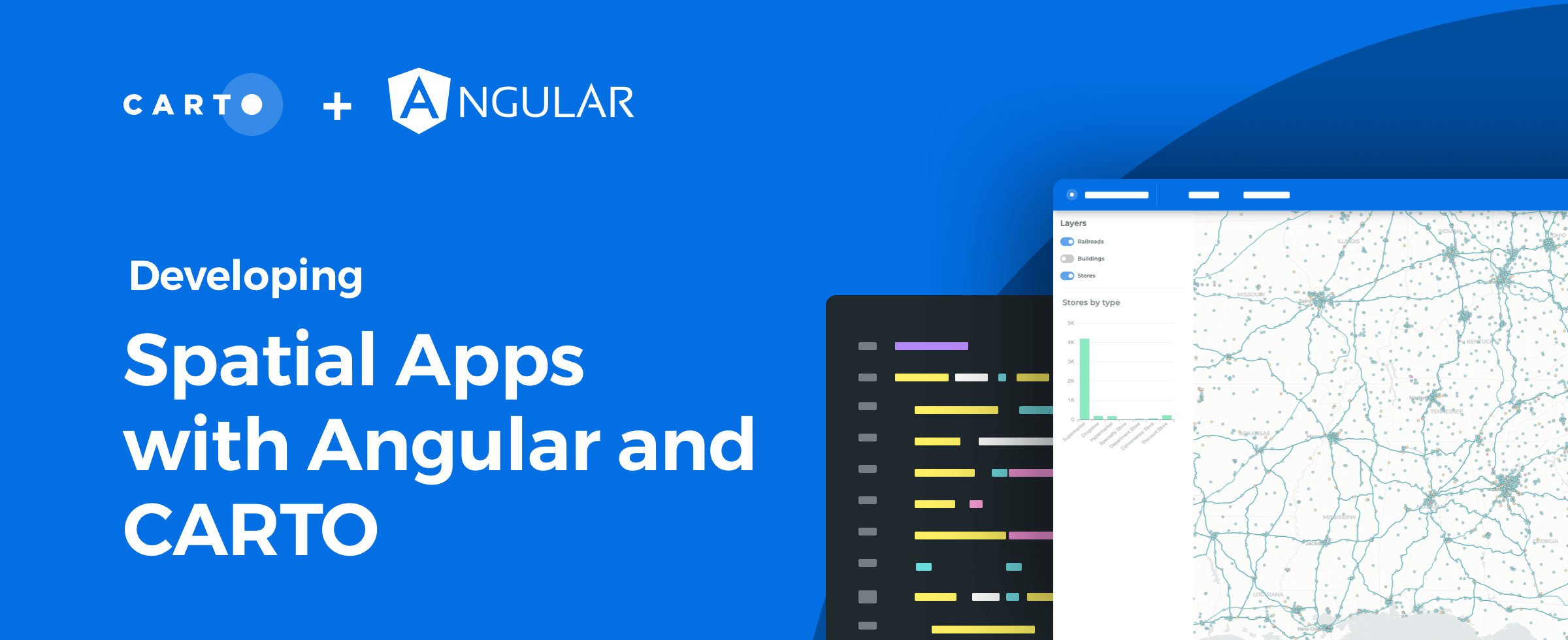
Angular is one of the most popular frameworks for building web applications with a huge community of developers. It includes powerful features for building scalable applications a great collection of libraries and tools for making developer’s lives easier.
If you want to build spatial applications CARTO + Angular is a really powerful combination. The CARTO platform provides many of the features you need for the backend of your spatial app so most of the time you just need to implement the frontend for your application.
Several CARTO customers and partners are building highly successful geospatial applications using Angular with our platform so we want to make sure we provide the best support to the community of Angular developers.
Our recommendation for building spatial web applications with CARTO is to use deck.gl as the visualization library. The CARTO for deck.gl module provides functionality for working with the CARTO platform and it is tightly integrated with our platform APIs.
We have created an example and an integration guide to show just how easy it is to integrate Angular applications with CARTO for deck.gl.
The example follows best practices for designing scalable and maintainable geospatial apps. These practices are the same we follow to build our own platform and our solutions like CARTO for React:
- Use of well supported open source libraries with a huge community behind them
- Reduced coupling among components with global state management
- Natural approach for experienced Angular developers
These are the main libraries used in this integration example:
- Angular Material for the user interface components
- Angular RxJS for managing communication/updates to components
- Angular Routing for page navigation
- Apache ECharts for charting
- Turf.js for client side spatial analysis
Our guide follows a step-by-step approach to building the example application from scratch with all the different modules services and components created using the Angular CLI.
Below you can see some code snippets to demonstrate how we have implemented this example.The common operations for working with layers (adding updating removing…) are centralized in a MapService. For example this is the implementation of the method for adding layer:
All the layers extend from the Layer class and need to implement the getLayer method that will return a deck.gl layer instance:
Communication between components takes advantage of the functionality offered by the RxJS library. For instance the MapService communicates viewstate updates using a BehaviorSubject and the chart component is subscribed to receive updates in order to recalculate the chart:
How to get started
If you want to get started building spatial apps with CARTO and Angular please check the guide. If you need additional information don’t hesitate to contact us.
Want to get started?